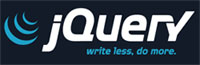
Normally using Jquery or Javascript to set focus on input box it will point to beginning of the text box. But if you want it point to last character in input text box. you may need write a Jquery class to do.
Below is the Jquery function:
(function($){ $.fn.focusTextToEnd = function(){ this.focus(); var $thisVal = this.val(); this.val('').val($thisVal); return this; } }(jQuery));
You may easy to use the function like below
$("#input").focusTextToEnd();
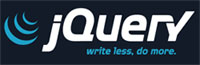
Jquery validation itself didn’t give money validate method function. What we can to do is write a custom method to validate the money format with regular expression.
Below is the money validate method.
jQuery.validator.addMethod( "money", function(value, element) { var isValidMoney = /^\d{0,4}(\.\d{0,2})?$/.test(value); return this.optional(element) || isValidMoney; }, "Insert " );
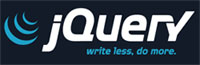
If want to check the specific Id already exists inside the site. you may using “length” to do it.
For Example:
if( $('#selector').length) { // it exists }if the length more then 0, that’s mean the following Id already exists inside the site.
if you are using class to check
if( $('.selector').length) { // it exists }
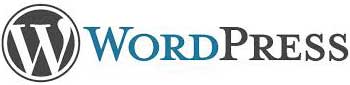
Below is the basic example of how to use AJAX in WordPress. It show you how to using javascript pass the variable to PHP function and then return back to javascript.
Javascript
var ajaxurl = '<?php echo admin_url() ?>admin-ajax.php'; jQuery(document).ready(function () { jQuery.ajax({ url: ajaxurl, data: { //This action must same with the PHP function name "action": "ajax_request", "params": "wordpress ajax" }, success:function(data) { //This is the result return from PHP function console.log(data); }, error: function(errorThrown){ //This is the error return console.log(errorThrown); } }); });
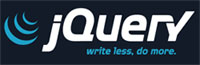
Below is the function I use to only number, delete, backspace and tap are allow.
$(document).on("keydown", "#number", function(e) { var key = e.charCode || e.keyCode || 0; if (key == 8 || key == 9 || key == 13 || key == 46 || key == 110 || key == 190 || (key >= 35 && key <= 40) ||(key >= 48 && key <= 57) || (key >= 96 && key <= 105)) { return; } else { return false; } });
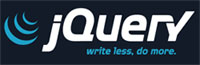
Dynamic dropdown options always use in our Application. It can be based on user first selection to return a new listing to second dropdown options by AJAX.
Below is the example PHP array script:
$option = array(); $option[] = array('name' => 'fruits', 'value' => 'f'); $option[] = array('name' => 'vegetables', 'value' => 'v'); $option[] = array('name' => 'pizza', 'value' => 'p');
And now we need the Jquery script to build the dropdown select. Below script are allow to reuse again when you need to replace the dropdown option again.
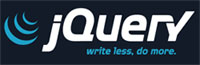
Below image was showing you display a div beside the mouse pointer or cursor. when user click the relative text.
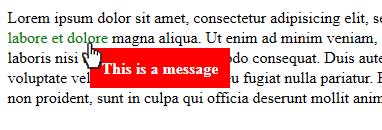
Click Here to check how the code work.
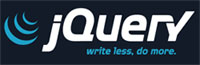
Jquery provide a lot of useful plugin. But sometimes you are allow using pure jQuery to complete the feature.
Below example code showing how to using pure jQuery to completed scroll to element feature.
JQuery smooth scroll example code
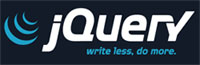
Jquery tablesorter is a powerful plugin for table sort and filter. It help me completed a lot of stuff.
But I found a problem, when I want filter by exact match results, the default filter function cannot do this. And I try add “filter-match” classes to the “th” header cells. Unfortunately the results return still same.
The Solution:
To filter by exact match, you may need modify or add “filter_functions” at the “widgetOptions“. The example: