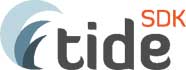
In here, I am showing you how to use TideSDK to display the phpinfo() and available php extension in your application.
First, use the
TideSDK Developer to create a new application. After that, open the
index.html file and edit the source.
In this example, I use JQuery to call the phpinfo(). So we need include the JQuery as well.
Include the JQuery inside the head.
<head>
<script src="jquery-2.0.1.min.js"></script>
</head>
We need include another PHP class to call the phpinfo() script to display the information. include the PHP file in head as well.
<head>
<script src="jquery-2.0.1.min.js"></script>
<script type="text/php" src="info.php"></script>
</head>
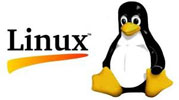
In Windows Machine if want to check our IP Address or Gateway the easy method is use Command Prompt type ipconfig then it will lists down everything. But in LINUX it different.
In Linux, they call it
ifconfig, open the terminal and type ifconfig it will lists down all the available network. But it didn’t print out the Gateway IP Address.
The easy way to print out Gateway IP Address command.
route -n | grep 'UG[ \t]' | awk '{print $2}'
Or, you can use others command to print out the Gateway.
Posted by
chevrons in
Linux
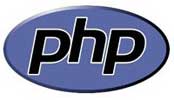
Here we describe how we can protect our class file that calling with include function, but it was not secure and its have big risk. For the solution if we can make the file cannot execute or calling when it not include by PHP file.
Why we need it? because when someone try calling http://localhost/module/foo.class.php it will be successful and maybe some accident will happen here.
if( basename( __FILE__ ) == basename( $_SERVER['PHP_SELF'] ) ) exit();
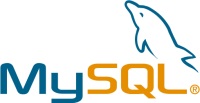
Duplicate row found and then update the row by one sql statement. This can be done by SQL, and not need any others server-side scripting.
Now let us see how it work. First, create table and insert some example data.
CREATE TABLE IF NOT EXISTS `foo` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(100) NOT NULL,
PRIMARY KEY (`id`),
UNIQUE KEY `name` (`name`)
);
INSERT INTO `foo` (`id`, `name`) VALUES
(1, 'foo'),
(2, 'foo2');
Posted by
chevrons in
MySQL
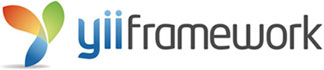
Below example showing you how to add the row number in cgridview widgets.
You just need add the following script inside the array
columns.
array(
'header' => 'No.',
'value' => '$this->grid->dataProvider->pagination->currentPage * $this->grid->dataProvider->pagination->pageSize + ($row+1)',
),
The completed example as below:
Posted by
chevrons in
Yii
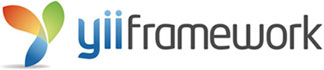
Normally we include Javascript in our application in Yii is below script
Yii::app()->getClientScript()->registerScriptFile('jquery.js');
But, now we need include yiigridview.js script file in our application. Because it was generate by Yii and it located at assets folder. So above example cannot used.
In here, we need to call
getAssetManager() to get the assets folder url and folder name generated by Yii.
Yii::app()->getAssetManager()->publish(Yii::getPathOfAlias('zii.widgets.assets'));
The above example will get the gridview path from the assets folder.
Posted by
chevrons in
Yii
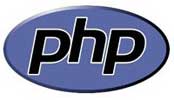
PHP shorthand is something that even if you don’t use it yourself, you’re sure to come across it elsewhere.
For Example:
if ($age > 12) {
$result = 'Young';
} else {
$result = 'Adult';
}
You can write it as:
$result = $age > 12 ? 'Young' : 'Adult';
And I also give some example at below:
Posted by
chevrons in
PHP
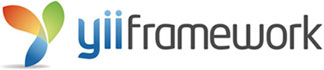
Following last post
CGridview listdata with CArrayDataProvider to list down the data with cgridview.
But it just can listing the records without the pagination. Use back last post example script and then modify the
CArrayDataProvider as below example.
Posted by
chevrons in
Yii
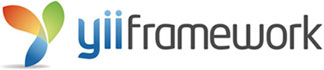
Yii, use CArrayDataProvider to listing out the array with CGridView widgets.
Below example are showing how to use it.
Data array:
$data = array();
$data[] = array('id' => 1, 'name' => 'name 1', 'age' => 22, 'date' => '1-Jan-2013');
$data[] = array('id' => 2, 'name' => 'name 2', 'age' => 25, 'date' => '1-Feb-2013');
$data[] = array('id' => 3, 'name' => 'name 3', 'age' => 22, 'date' => '1-Mac-2013');
$data[] = array('id' => 4, 'name' => 'name 4', 'age' => 28, 'date' => '1-Jan-2013');
$data[] = array('id' => 5, 'name' => 'name 5', 'age' => 23, 'date' => '1-Apr-2013');
$data[] = array('id' => 6, 'name' => 'name 6', 'age' => 29, 'date' => '1-May-2013');
$data[] = array('id' => 7, 'name' => 'name 7', 'age' => 21, 'date' => '1-Jan-2013');
$data[] = array('id' => 8, 'name' => 'name 8', 'age' => 20, 'date' => '1-Feb-2013');
Posted by
chevrons in
Yii
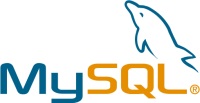
The easiest way to generate random records in Mysql is to use the
ORDER BY RAND().
SELECT col1 FROM tbl ORDER BY RAND() LIMIT 1;
This can work fine for small tables. However, for big table, it will have a serious performance problem as in order to generate the list of random rows, because Mysql need to assign random number to each row and then sort them.
Even if you only want random 1 records from 200k+ rows, Mysql need to sort all the records and then extract it.
Posted by
chevrons in
MySQL